ell::SPC_MinPair Class Reference
#include <StatePairCollector.hh>
Inheritance diagram for ell::SPC_MinPair:
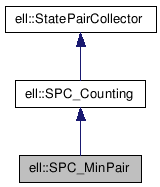
Detailed Description
This class is used to keep track of the number of States pairs added to the collector and stores the minimal pair added.A base pair (i,j) is considered to be smaller than another (k,l) if max(i,j) < max(k,l) according to the State::less operator.
Definition at line 281 of file StatePairCollector.hh.
Public Types | |
typedef std::pair< const State *, const State * > | StatePair |
Public Member Functions | |
virtual void | add (const StatePair &s)=0 |
virtual void | add (const State *const s1, const State *const s2) |
virtual void | add (const StatePair &s) |
virtual const StatePair | getLastAdded () const |
virtual const StatePair | getMinPair () const |
virtual size_t | size () const |
SPC_MinPair (const bool pairsAddedInAscOrder=false) | |
virtual | ~SPC_MinPair () |
Protected Member Functions | |
void | checkMinPair () |
void | clearLastAdded () |
void | clearMinPair () |
Protected Attributes | |
State * | lastAdded_s1 |
State * | lastAdded_s2 |
const State * | minPair_max |
State * | minPair_s1 |
State * | minPair_s2 |
const bool | pairsAddedInAscOrder |
size_t | statePairCount |
Member Typedef Documentation
typedef std::pair<const State*,const State*> ell::StatePairCollector::StatePair [inherited] |
Constructor & Destructor Documentation
ell::SPC_MinPair::SPC_MinPair | ( | const bool | pairsAddedInAscOrder = false |
) |
Construction. Whether or not the pairs are added to the collector in ascending order such that the first reported pair is the minimal one. This reduces the comparison effort enormous!
Definition at line 189 of file StatePairCollector.cc.
ell::SPC_MinPair::~SPC_MinPair | ( | ) | [virtual] |
Definition at line 198 of file StatePairCollector.cc.
Member Function Documentation
virtual void ell::StatePairCollector::add | ( | const StatePair & | s | ) | [pure virtual, inherited] |
Increments State pair counter and stores this pair as the last added.
Reimplemented from ell::SPC_Counting.
Definition at line 217 of file StatePairCollector.cc.
void ell::SPC_MinPair::add | ( | const StatePair & | s | ) | [virtual] |
Increments State pair counter and stores a copy this pair as the last added.
- Parameters:
-
s the State pair to add
Reimplemented from ell::SPC_Counting.
Definition at line 207 of file StatePairCollector.cc.
void ell::SPC_MinPair::checkMinPair | ( | ) | [protected] |
checks if the last added state pair is smaller than the current
Definition at line 234 of file StatePairCollector.cc.
void ell::SPC_Counting::clearLastAdded | ( | ) | [inline, protected, inherited] |
void ell::SPC_MinPair::clearMinPair | ( | ) | [inline, protected] |
const SPC_Counting::StatePair ell::SPC_Counting::getLastAdded | ( | ) | const [virtual, inherited] |
Returns last added State pair. Note: The pointed States will be deleted when the collector is destroyed. So a cloning is needed in case the pair will be needed later.
- Returns:
- last added State pair
Implements ell::StatePairCollector.
Definition at line 61 of file StatePairCollector.cc.
const SPC_Counting::StatePair ell::SPC_MinPair::getMinPair | ( | ) | const [virtual] |
Returns minimal pair of States added. Note: The pointed States will be deleted when the collector is destroyed. So a cloning is needed in case the pair will be needed later.
- Returns:
- minimal added State pair
Definition at line 229 of file StatePairCollector.cc.
size_t ell::SPC_Counting::size | ( | ) | const [virtual, inherited] |
Returns number of added State pairs.
- Returns:
- the number of added state pairs
Implements ell::StatePairCollector.
Definition at line 54 of file StatePairCollector.cc.
Field Documentation
State* ell::SPC_Counting::lastAdded_s1 [protected, inherited] |
State* ell::SPC_Counting::lastAdded_s2 [protected, inherited] |
const State* ell::SPC_MinPair::minPair_max [protected] |
pointer to the higher of both minPair states according to State::less operator
Definition at line 297 of file StatePairCollector.hh.
State* ell::SPC_MinPair::minPair_s1 [protected] |
the first State of the minimal added State pair
Definition at line 292 of file StatePairCollector.hh.
State* ell::SPC_MinPair::minPair_s2 [protected] |
the second State of the minimal added State pair
Definition at line 294 of file StatePairCollector.hh.
const bool ell::SPC_MinPair::pairsAddedInAscOrder [protected] |
Whether or not the pairs are added to the collector in ascending order such that the first reported pair is the minimal one. This reduces the comparison effort enormous!
Definition at line 289 of file StatePairCollector.hh.
size_t ell::SPC_Counting::statePairCount [protected, inherited] |
The documentation for this class was generated from the following files:
- src/ell/StatePairCollector.hh
- src/ell/StatePairCollector.cc