ell::S_SG_Ising Class Reference
#include <S_SG_Ising.hh>
Inheritance diagram for ell::S_SG_Ising:
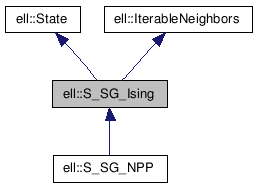
Detailed Description
Representing a connected ring or spins.Spins are neighbored iif : i+1 == j or (i==0 and j==(spinnumber-1))
Definition at line 17 of file S_SG_Ising.hh.
Public Types | |
typedef biu::VirtualList< State > | NeighborList |
typedef std::auto_ptr< NeighborList > | NeighborListPtr |
typedef biu::Alphabet::Sequence | SpinArray |
typedef biu::Alphabet::AlphElem | SpinType |
Public Member Functions | |
virtual State * | applyNeighborChange (const size_t index, State *const neigh) const |
virtual S_SG_Ising * | clone (State *toFill=NULL) const |
virtual CSequence & | compress (CSequence &toFill) const |
virtual CSequence | compress (void) const |
virtual State * | fromString (const std::string &stringRep) const |
virtual double | getEnergy () const |
virtual const std::string & | getID (void) const |
virtual unsigned int | getMinimalDistance (const State &state2) const |
virtual State * | getNeighbor (const size_t index, State *neigh) const |
virtual NeighborListPtr | getNeighborList () const |
virtual size_t | getNeighborNumber (void) const |
virtual State * | getRandomNeighbor (State *inPlaceNeigh=NULL) const |
virtual NeighborListPtr | getRandomNeighborList () const |
virtual bool | operator!= (const State &state2) const |
virtual bool | operator< (const State &state2) const |
virtual void | operator= (const S_SG_Ising &sg2) |
virtual bool | operator== (const State &state2) const |
S_SG_Ising (const S_SG_Ising &s2) | |
S_SG_Ising (const std::string &spinStr) | |
S_SG_Ising (size_t size, bool random=true) | |
virtual std::string & | toString (std::string &toFill) const |
virtual std::string | toString () const |
virtual State * | uncompress (const CSequence &cseq) |
virtual State * | uncompress (const CSequence &cseq, State *toFill) const |
virtual State * | undoNeighborChange (const size_t index, State *const neigh) const |
virtual | ~S_SG_Ising () |
Static Public Member Functions | |
static bool | less (const State *s1, const State *s2) |
Static Public Attributes | |
static const std::string | ID |
static const SpinType | SPIN_DOWN |
static const SpinType | SPIN_UP |
Protected Member Functions | |
SpinType | flipSpin (SpinType s) const |
Protected Attributes | |
SpinArray | spin |
Static Protected Attributes | |
static const biu::Alphabet | spinAlph |
Member Typedef Documentation
typedef biu::VirtualList<State> ell::State::NeighborList [inherited] |
typedef std::auto_ptr<NeighborList> ell::State::NeighborListPtr [inherited] |
typedef biu::Alphabet::Sequence ell::S_SG_Ising::SpinArray |
typedef biu::Alphabet::AlphElem ell::S_SG_Ising::SpinType |
Constructor & Destructor Documentation
ell::S_SG_Ising::S_SG_Ising | ( | size_t | size, | |
bool | random = true | |||
) |
Constructs a spin array of the given size and initializes with SPIN_UP for all spins or a random distribution.
- Parameters:
-
size the size of the spin array random if true a random initialization is done
Definition at line 20 of file S_SG_Ising.cc.
ell::S_SG_Ising::S_SG_Ising | ( | const std::string & | spinStr | ) |
Constructs a spin array from the given spin string
- Parameters:
-
spinStr the +- spin string
Definition at line 30 of file S_SG_Ising.cc.
ell::S_SG_Ising::S_SG_Ising | ( | const S_SG_Ising & | s2 | ) |
Definition at line 37 of file S_SG_Ising.cc.
ell::S_SG_Ising::~S_SG_Ising | ( | ) | [virtual] |
Definition at line 42 of file S_SG_Ising.cc.
Member Function Documentation
State * ell::S_SG_Ising::applyNeighborChange | ( | const size_t | index, | |
State *const | neigh | |||
) | const [virtual] |
Applies the necessary changes to a given state to get the neighbor. Note: neigh is expected to be a copy (i.e. *this == *neigh) and therefore direct changes are possible or to be NULL. If (neigh==NULL) a copy of this has to be generated, modified, and returned.
- Parameters:
-
index the neighbor to generate neigh a copy of this the changes should be applied to
- Returns:
- the neighbor state of the given index or NULL if the index is out of range
Implements ell::IterableNeighbors.
Reimplemented in ell::S_SG_NPP.
Definition at line 238 of file S_SG_Ising.cc.
S_SG_Ising * ell::S_SG_Ising::clone | ( | State * | toFill = NULL |
) | const [virtual] |
Returns a pointer to a clone of the current state.
- Returns:
- a pointer to a copy of this state, if toFill is != NULL the return value corresponds to the updated state pointed to by toFill, otherwise a new object is created !!!
Implements ell::State.
Reimplemented in ell::S_SG_NPP.
Definition at line 107 of file S_SG_Ising.cc.
Access to a compressed sequence representation of the state.
- Parameters:
-
toFill a data structure to write the compressed representation too
- Returns:
- the compressed sequence representation
Implements ell::State.
Definition at line 265 of file S_SG_Ising.cc.
CSequence ell::S_SG_Ising::compress | ( | void | ) | const [virtual] |
Access to a compressed sequence representation of the state.
- Returns:
- the compressed sequence representation
Implements ell::State.
Definition at line 254 of file S_SG_Ising.cc.
State * ell::S_SG_Ising::fromString | ( | const std::string & | stringRep | ) | const [virtual] |
Returns a new State based on the current state. The new state differs only by the information given by stringRep.
Implements ell::State.
Reimplemented in ell::S_SG_NPP.
Definition at line 125 of file S_SG_Ising.cc.
double ell::S_SG_Ising::getEnergy | ( | ) | const [virtual] |
Returns the state specific energy, i.e. E = sum ( J(i,j)*spin(i)*spin(j) ) for all 0<=i<j<=spinnumber and J(i,j) = 1 iif spin i and j are neighbored and 0 otherwise.
Implements ell::State.
Reimplemented in ell::S_SG_NPP.
Definition at line 75 of file S_SG_Ising.cc.
const std::string & ell::S_SG_Ising::getID | ( | void | ) | const [virtual] |
Access to a State subclass specific ID string to identify instances of this class.
- Returns:
- the subclass specific ID string
Implements ell::State.
Reimplemented in ell::S_SG_NPP.
Definition at line 47 of file S_SG_Ising.cc.
unsigned int ell::S_SG_Ising::getMinimalDistance | ( | const State & | state2 | ) | const [virtual] |
Returns the minimal number of steps via valid neighbored states from this to another valid State.
- Parameters:
-
state2 the State to reach
- Returns:
- if state2 is a S_SG_Ising state the minimal distance or UINT_MAX otherwise
Implements ell::State.
Definition at line 92 of file S_SG_Ising.cc.
Access to a specific neighbor.
- Parameters:
-
index the index of the neighbor in [0, getNeighborNumber()) neigh if != NULL this state should be converted into the neighbor otherwise a new one is created and returned
- Returns:
- the new neighbor state or NULL if the index is >= getNeighborNumber()
Implements ell::IterableNeighbors.
Reimplemented in ell::S_SG_NPP.
Definition at line 199 of file S_SG_Ising.cc.
State::NeighborListPtr ell::S_SG_Ising::getNeighborList | ( | ) | const [virtual] |
Returns a virtual list of all VALID neighbored states in the energy landscape in a specific successive order.
Implements ell::State.
Definition at line 148 of file S_SG_Ising.cc.
size_t ell::S_SG_Ising::getNeighborNumber | ( | void | ) | const [virtual] |
How many neighbor indices are accessible.
- Returns:
- the number of neighbors
Implements ell::IterableNeighbors.
Definition at line 188 of file S_SG_Ising.cc.
Returns a VALID random neighbored state. If a state is given it is overwritten and changed to a neighbor.
Implements ell::State.
Reimplemented in ell::S_SG_NPP.
Definition at line 165 of file S_SG_Ising.cc.
State::NeighborListPtr ell::S_SG_Ising::getRandomNeighborList | ( | ) | const [virtual] |
Returns a virtual list of all VALID neighbored states in the energy landscape in a random order.
Implements ell::State.
Definition at line 157 of file S_SG_Ising.cc.
Comparison function that compares two State pointer based on the less than operator '<' of the first State. The function can be used in STL algorithms, e.g. if only State pointer are stored but the ordering should be based on the object order.
- Parameters:
-
s1 the State pointer of the object that is asked to be smaller (!=NULL) s2 the State pointer of the object that is asked to be bigger (!=NULL)
- Returns:
- s1->operator <(*s1);
Reimplemented in ell::S_Explicit.
bool ell::S_SG_Ising::operator!= | ( | const State & | state2 | ) | const [virtual] |
bool ell::State::operator< | ( | const State & | state2 | ) | const [inline, virtual, inherited] |
Implements a unique order on states based on their energy and string representation. A state is smaller than another one iff it has smaller energy or it has equal energy and a lexicographically smaller string representation (tie breaker).
This function will be overwritten to achive a better performance than calling getEnergy() and getString().
If the energy function is non-degenerate the string comparison is obsolete.
- Parameters:
-
state2 the State object to compare to
- Returns:
- true if this state is smaller than state2 according to the unique order of the states
Reimplemented in ell::S_LP, ell::S_RNAfe_SingleM, ell::S_RNAfe_SingleM_TB, and ell::S_Explicit.
void ell::S_SG_Ising::operator= | ( | const S_SG_Ising & | sg2 | ) | [virtual] |
Definition at line 62 of file S_SG_Ising.cc.
bool ell::S_SG_Ising::operator== | ( | const State & | state2 | ) | const [virtual] |
std::string & ell::S_SG_Ising::toString | ( | std::string & | toFill | ) | const [virtual] |
Fills the given string with a specific std::string representation of this State.
- Parameters:
-
toFill the string to overwrite
- Returns:
- the changed toFill in parameter
Implements ell::State.
Definition at line 138 of file S_SG_Ising.cc.
std::string ell::S_SG_Ising::toString | ( | ) | const [virtual] |
Returns a +- std::string representation of this spin glass array.
Implements ell::State.
Definition at line 133 of file S_SG_Ising.cc.
Uncompresses a compressed sequencce representation into a this State object.
- Parameters:
-
cseq the compressed sequence representation of a state
- Returns:
- this or NULL in error case
Implements ell::State.
Definition at line 298 of file S_SG_Ising.cc.
Uncompresses a compressed sequencce representation into a new State object.
- Parameters:
-
cseq the compressed sequence representation of a state toFill a state object to uncompress too or NULL if a new object has to be created
- Returns:
- new State object that is encoded in cseq or NULL in error case.
Implements ell::State.
Reimplemented in ell::S_SG_NPP.
Definition at line 280 of file S_SG_Ising.cc.
State * ell::S_SG_Ising::undoNeighborChange | ( | const size_t | index, | |
State *const | neigh | |||
) | const [virtual] |
Undo the changes done to this state to generate the given neighbor. The goal is to do less operations than doing a full copy like (*neigh = *this).
- Parameters:
-
index the neighbor index that was applied last [ 0, getNeighborNumber() ) neigh the state resulting from the last changes ( != NULL)
- Returns:
- the copy of this object via undoing the last changes in neigh
Implements ell::IterableNeighbors.
Definition at line 220 of file S_SG_Ising.cc.
Field Documentation
const std::string ell::S_SG_Ising::ID [static] |
the specific ID string of this class
Reimplemented in ell::S_SG_NPP.
Definition at line 32 of file S_SG_Ising.hh.
SpinArray ell::S_SG_Ising::spin [protected] |
const S_SG_Ising::SpinType ell::S_SG_Ising::SPIN_DOWN [static] |
const S_SG_Ising::SpinType ell::S_SG_Ising::SPIN_UP [static] |
const biu::Alphabet ell::S_SG_Ising::spinAlph [static, protected] |
The documentation for this class was generated from the following files:
- src/ell/spinglass/S_SG_Ising.hh
- src/ell/spinglass/S_SG_Ising.cc