biu::SquareMatrix< T, matrixDim > Class Template Reference
#include <SquareMatrix.hh>
Inheritance diagram for biu::SquareMatrix< T, matrixDim >:
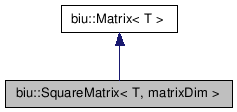
Detailed Description
template<class T, size_t matrixDim>
class biu::SquareMatrix< T, matrixDim >
Generic square matrix with several matrix operations.
Definition at line 14 of file SquareMatrix.hh.
Public Member Functions | |
T & | at (const size_t r, const size_t c) |
T | at (const size_t r, const size_t c) const |
std::vector< T > | columnVec (const size_t col) const |
size_t | numColumns () const |
size_t | numRows () const |
std::vector< T > | operator * (const std::vector< T > &vec) const |
Matrix< T > | operator * (const T &c) const |
Matrix< T > | operator * (const Matrix< T > &mat) const |
Matrix< T > & | operator *= (const T &c) |
Matrix< T > & | operator *= (const Matrix< T > &mat) |
bool | operator!= (const T &val) const |
bool | operator!= (const Matrix< T > &mat) const |
Matrix< T > | operator+ (const T &c) |
Matrix< T > | operator+ (const Matrix< T > &mat) |
Matrix< T > | operator- (const T &c) |
Matrix< T > | operator- (const Matrix< T > &mat) |
bool | operator== (const T &val) const |
bool | operator== (const Matrix< T > &mat) const |
const T *const | operator[] (const size_t row) const |
T *const | operator[] (const size_t row) |
void | resize (const size_t row, const size_t col, const T &defVal) |
void | resize (const size_t row, const size_t col) |
SquareMatrix (const T m[matrixDim][matrixDim]) | |
SquareMatrix (const Matrix< T > &m) | |
SquareMatrix (const SquareMatrix &m) | |
SquareMatrix () | |
~SquareMatrix () | |
Static Public Member Functions | |
static SquareMatrix< T, matrixDim > | createSquareMatrix (const T data[matrixDim][matrixDim]) |
Protected Attributes | |
size_t | cols |
size_t | rows |
T ** | v |
Constructor & Destructor Documentation
biu::SquareMatrix< T, matrixDim >::SquareMatrix | ( | ) | [inline] |
biu::SquareMatrix< T, matrixDim >::SquareMatrix | ( | const SquareMatrix< T, matrixDim > & | m | ) | [inline] |
biu::SquareMatrix< T, matrixDim >::SquareMatrix | ( | const Matrix< T > & | m | ) | [inline] |
biu::SquareMatrix< T, matrixDim >::SquareMatrix | ( | const T | m[matrixDim][matrixDim] | ) | [inline] |
biu::SquareMatrix< T, matrixDim >::~SquareMatrix | ( | ) | [inline] |
Definition at line 70 of file SquareMatrix.hh.
Member Function Documentation
T& biu::Matrix< T >::at | ( | const size_t | r, | |
const size_t | c | |||
) | [inline, inherited] |
direct access to element (r,c)
- Parameters:
-
r the row to access c the column to access
- Returns:
- the matrix element at position (r,c)
T biu::Matrix< T >::at | ( | const size_t | r, | |
const size_t | c | |||
) | const [inline, inherited] |
constant access to element (r,c)
- Parameters:
-
r the row to access c the column to access
- Returns:
- the matrix element at position (r,c)
std::vector<T> biu::Matrix< T >::columnVec | ( | const size_t | col | ) | const [inherited] |
access to a matrix column
- Parameters:
-
col the column to access
- Returns:
- the column vector
SquareMatrix< T, matrixDim > biu::SquareMatrix< T, matrixDim >::createSquareMatrix | ( | const T | data[matrixDim][matrixDim] | ) | [inline, static] |
Definition at line 78 of file SquareMatrix.hh.
size_t biu::Matrix< T >::numColumns | ( | ) | const [inline, inherited] |
number of columns
- Returns:
- column number
size_t biu::Matrix< T >::numRows | ( | ) | const [inline, inherited] |
number of rows
- Returns:
- row number
std::vector<T> biu::Matrix< T >::operator * | ( | const std::vector< T > & | vec | ) | const [inherited] |
special case of * column number has to equal vec size
- Parameters:
-
vec the column vector to multiply with
- Returns:
- a new column vector representing (this * vec)
Matrix<T> biu::Matrix< T >::operator * | ( | const T & | c | ) | const [inherited] |
matrix * constant
- Parameters:
-
c the constant to multiply all elements with
- Returns:
- a new matrix representing (this * c)
Matrix<T> biu::Matrix< T >::operator * | ( | const Matrix< T > & | mat | ) | const [inherited] |
matrix * matrix row number have to equal column number and vice versa
- Parameters:
-
mat the matrix to multiply with
- Returns:
- a new matrix representing (this * mat)
Matrix<T>& biu::Matrix< T >::operator *= | ( | const T & | c | ) | [inherited] |
matrix *= constant
- Parameters:
-
c the constant to multiply all elements with
- Returns:
- *this
Matrix<T>& biu::Matrix< T >::operator *= | ( | const Matrix< T > & | mat | ) | [inherited] |
matrix *= matrix row number have to equal column number and vice versa
- Parameters:
-
mat the matrix to multiply with
- Returns:
- *this
bool biu::Matrix< T >::operator!= | ( | const T & | val | ) | const [inline, inherited] |
test if at least one element doesnt equal val
- Parameters:
-
val the value to compare with
- Returns:
- true if at least one element are is not equal, false otherwise
bool biu::Matrix< T >::operator!= | ( | const Matrix< T > & | mat | ) | const [inline, inherited] |
test for inequality
- Parameters:
-
mat the matrix to compare with
- Returns:
- true if dimensions or at least one element are is not equal, false otherwise
Matrix<T> biu::Matrix< T >::operator+ | ( | const T & | c | ) | [inherited] |
matrix + constant
- Parameters:
-
c the constant to add to all elements
- Returns:
- a new matrix representing (this + c)
Matrix<T> biu::Matrix< T >::operator+ | ( | const Matrix< T > & | mat | ) | [inherited] |
matrix + matrix row and column number have to be equal
- Parameters:
-
mat the matrix to add
- Returns:
- a new matrix representing (this + mat)
Matrix<T> biu::Matrix< T >::operator- | ( | const T & | c | ) | [inherited] |
matrix - constant
- Parameters:
-
c the constant to substract from all elements
- Returns:
- a new matrix representing (this - c)
Matrix<T> biu::Matrix< T >::operator- | ( | const Matrix< T > & | mat | ) | [inherited] |
matrix - matrix row and column number have to be equal
- Parameters:
-
mat the matrix to substract
- Returns:
- a new matrix representing (this - mat)
bool biu::Matrix< T >::operator== | ( | const T & | val | ) | const [inline, inherited] |
test if all elements == val
- Parameters:
-
val the value to compare with
- Returns:
- true if all elements are equal, false otherwise
bool biu::Matrix< T >::operator== | ( | const Matrix< T > & | mat | ) | const [inline, inherited] |
test for equality
- Parameters:
-
mat the matrix to compare with
- Returns:
- true if dimensions and all elements are equal, false otherwise
const T* const biu::Matrix< T >::operator[] | ( | const size_t | row | ) | const [inline, inherited] |
constant access to row i
- Parameters:
-
row the row to access
- Returns:
- constant array access to the row
T* const biu::Matrix< T >::operator[] | ( | const size_t | row | ) | [inline, inherited] |
access to row
- Parameters:
-
row the row to access
- Returns:
- array access to the row
void biu::Matrix< T >::resize | ( | const size_t | row, | |
const size_t | col, | |||
const T & | defVal | |||
) | [inherited] |
Resizes the matrix. If the the dimensions are increased, the new fields are filled with the given default value
- Parameters:
-
row the new row number col the new column number defVal the default value for new matrix elements
void biu::Matrix< T >::resize | ( | const size_t | row, | |
const size_t | col | |||
) | [inherited] |
Resizes the matrix. If the the dimensions are increased, the new fields are not initialised.
- Parameters:
-
row the new row number col the new column number
Field Documentation
size_t biu::Matrix< T >::cols [protected, inherited] |
size_t biu::Matrix< T >::rows [protected, inherited] |
T** biu::Matrix< T >::v [protected, inherited] |
The documentation for this class was generated from the following file:
- src/biu/SquareMatrix.hh