ell::State Class Reference
#include <State.hh>
Inheritance diagram for ell::State:
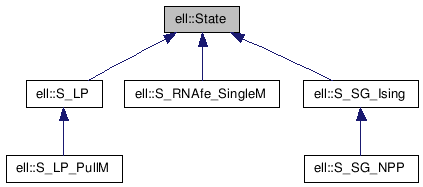
Detailed Description
An object of the class State represents a state in the energy landscape. It handles its fitness and neighborhood concerning the landscape.A State is always valid in the subclass.
Definition at line 36 of file State.hh.
Public Types | |
typedef biu::VirtualList< State > | NeighborList |
typedef std::auto_ptr< NeighborList > | NeighborListPtr |
Public Member Functions | |
virtual State * | clone () const=0 |
virtual CSequence & | compress (CSequence &toFill) const=0 |
virtual CSequence | compress (void) const =0 |
virtual State * | fromString (const std::string &stringRep) const =0 |
virtual double | getEnergy () const=0 |
virtual int | getFitness () const=0 |
virtual unsigned int | getMinimalDistance (const State &state2) const =0 |
virtual NeighborListPtr | getNeighborList () const=0 |
virtual State * | getRandomNeighbor (State *inPlaceNeigh=NULL) const=0 |
virtual NeighborListPtr | getRandomNeighborList () const=0 |
virtual bool | operator!= (const State &state2) const =0 |
virtual bool | operator== (const State &state2) const =0 |
State () | |
virtual std::string | toString () const=0 |
virtual State * | uncompress (const CSequence &cseq)=0 |
virtual State * | uncompress (const CSequence &cseq, State *toFill) const =0 |
virtual | ~State () |
Member Typedef Documentation
typedef biu::VirtualList<State> ell::State::NeighborList |
typedef std::auto_ptr<NeighborList> ell::State::NeighborListPtr |
Constructor & Destructor Documentation
Member Function Documentation
virtual State* ell::State::clone | ( | ) | const [pure virtual] |
Returns a pointer to a clone of the current state.
Implemented in ell::S_LP, ell::S_LP_PullM, ell::S_RNAfe_SingleM, ell::S_SG_Ising, and ell::S_SG_NPP.
Access to a compressed sequence representation of the state.
- Parameters:
-
toFill a data structure to write the compressed representation too
- Returns:
- the compressed sequence representation
Implemented in ell::S_LP, ell::S_LP_PullM, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
virtual CSequence ell::State::compress | ( | void | ) | const [pure virtual] |
Access to a compressed sequence representation of the state.
- Returns:
- the compressed sequence representation
Implemented in ell::S_LP, ell::S_LP_PullM, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
virtual State* ell::State::fromString | ( | const std::string & | stringRep | ) | const [pure virtual] |
Returns a new State based on the current state. The new state differs only by the information given by stringRep.
Implemented in ell::S_LP, ell::S_LP_PullM, ell::S_RNAfe_SingleM, ell::S_SG_Ising, and ell::S_SG_NPP.
virtual double ell::State::getEnergy | ( | ) | const [pure virtual] |
Returns the state specific energy.
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, ell::S_SG_Ising, and ell::S_SG_NPP.
virtual int ell::State::getFitness | ( | ) | const [pure virtual] |
Returns the state specific fitness.
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
virtual unsigned int ell::State::getMinimalDistance | ( | const State & | state2 | ) | const [pure virtual] |
Returns the minimal number of steps via valid neighbored states from this to another valid State.
- Parameters:
-
state2 the State to reach
Implemented in ell::S_LP_PullM, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
virtual NeighborListPtr ell::State::getNeighborList | ( | ) | const [pure virtual] |
Returns a virtual list of all VALID neighbored states in the energy landscape in a specific order.
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
Returns a VALID random neighbored state. If a state is given, this is changed to a neighbor.
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, ell::S_SG_Ising, and ell::S_SG_NPP.
virtual NeighborListPtr ell::State::getRandomNeighborList | ( | ) | const [pure virtual] |
Returns a virtual list of all VALID neighbored states in the energy landscape in a random order. If a state is given, this is changed to a neighbor.
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
virtual bool ell::State::operator!= | ( | const State & | state2 | ) | const [pure virtual] |
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, ell::S_SG_Ising, and ell::S_SG_NPP.
virtual bool ell::State::operator== | ( | const State & | state2 | ) | const [pure virtual] |
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, ell::S_SG_Ising, and ell::S_SG_NPP.
virtual std::string ell::State::toString | ( | ) | const [pure virtual] |
Returns a specific std::string representation of this State.
Implemented in ell::S_LP, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
Uncompresses a compressed sequencce representation into a this State object.
- Parameters:
-
cseq the compressed sequence representation of a state
- Returns:
- this or NULL in error case
Implemented in ell::S_LP, ell::S_LP_PullM, ell::S_RNAfe_SingleM, and ell::S_SG_Ising.
virtual State* ell::State::uncompress | ( | const CSequence & | cseq, | |
State * | toFill | |||
) | const [pure virtual] |
Uncompresses a compressed sequencce representation into a new State object.
- Parameters:
-
cseq the compressed sequence representation of a state toFill a state object to uncompress too or NULL if a new object has to be created
- Returns:
- new State object that is encoded in cseq or NULL in error case.
Implemented in ell::S_LP, ell::S_LP_PullM, ell::S_RNAfe_SingleM, ell::S_SG_Ising, and ell::S_SG_NPP.
The documentation for this class was generated from the following file:
- src/ell/State.hh