biu::Point3D< T > Class Template Reference
#include <Point.hh>
Inheritance diagram for biu::Point3D< T >:
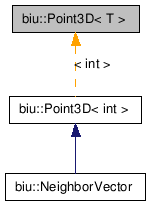
Detailed Description
template<class T>
class biu::Point3D< T >
The template Point3D represents a point in 3-dimensional space for different base types.
Definition at line 41 of file Point.hh.
Public Member Functions | |
double | distance (const Point3D &p2) const |
T | getX () const |
T | getY () const |
T | getZ () const |
bool | isEven () const |
Point3D & | operator *= (const T &d) |
operator Point3D () const | |
operator std::vector () const | |
bool | operator!= (const Point3D &p2) const |
Point3D | operator+ (const Point3D &p2) const |
Point3D & | operator+= (const T &d) |
Point3D & | operator+= (const Point3D &p2) |
Point3D & | operator- () |
Point3D | operator- (const Point3D &p2) const |
Point3D & | operator-= (const T &d) |
Point3D & | operator-= (const Point3D &p2) |
Point3D & | operator/= (const T &d) |
bool | operator< (const Point3D &p2) const |
Point3D & | operator= (const Point3D &p2) |
bool | operator== (const Point3D &p2) const |
bool | operator> (const Point3D &p2) const |
Point3D (const std::vector< T > &v) | |
Point3D (const Point3D &p2) | |
Point3D (const T _x, const T _y, const T _z) | |
Point3D () | |
void | setX (const T &x_) |
void | setY (const T &y_) |
void | setZ (const T &z_) |
double | vectorLength () const |
~Point3D () | |
Private Member Functions | |
template<int > | |
bool | equal (const int &a, const int &b) const |
bool | equal (const T &a, const T &b) const |
Private Attributes | |
T | x |
T | y |
T | z |
Friends | |
std::ostream & | operator<< (std::ostream &out, const Point3D &p) |
Constructor & Destructor Documentation
template<class T>
biu::Point3D< T >::Point3D | ( | ) | [inline] |
template<class T>
biu::Point3D< T >::Point3D | ( | const T | _x, | |
const T | _y, | |||
const T | _z | |||
) | [inline] |
template<class T>
biu::Point3D< T >::Point3D | ( | const Point3D< T > & | p2 | ) | [inline] |
template<class T>
biu::Point3D< T >::Point3D | ( | const std::vector< T > & | v | ) | [inline] |
template<class T>
biu::Point3D< T >::~Point3D | ( | ) | [inline] |
Member Function Documentation
template<class T>
double biu::Point3D< T >::distance | ( | const Point3D< T > & | p2 | ) | const [inline] |
template<class T>
template<int >
bool biu::Point3D< T >::equal | ( | const int & | a, | |
const int & | b | |||
) | const [inline, private] |
template<class T>
bool biu::Point3D< T >::equal | ( | const T & | a, | |
const T & | b | |||
) | const [inline, private] |
template<class T>
T biu::Point3D< T >::getX | ( | ) | const [inline] |
template<class T>
T biu::Point3D< T >::getY | ( | ) | const [inline] |
template<class T>
T biu::Point3D< T >::getZ | ( | ) | const [inline] |
template<class T>
bool biu::Point3D< T >::isEven | ( | ) | const [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator *= | ( | const T & | d | ) | [inline] |
template<class T>
biu::Point3D< T >::operator Point3D | ( | ) | const [inline] |
template<class T>
biu::Point3D< T >::operator std::vector | ( | ) | const [inline] |
template<class T>
bool biu::Point3D< T >::operator!= | ( | const Point3D< T > & | p2 | ) | const [inline] |
template<class T>
Point3D biu::Point3D< T >::operator+ | ( | const Point3D< T > & | p2 | ) | const [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator+= | ( | const T & | d | ) | [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator+= | ( | const Point3D< T > & | p2 | ) | [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator- | ( | ) | [inline] |
template<class T>
Point3D biu::Point3D< T >::operator- | ( | const Point3D< T > & | p2 | ) | const [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator-= | ( | const T & | d | ) | [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator-= | ( | const Point3D< T > & | p2 | ) | [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator/= | ( | const T & | d | ) | [inline] |
template<class T>
bool biu::Point3D< T >::operator< | ( | const Point3D< T > & | p2 | ) | const [inline] |
template<class T>
Point3D& biu::Point3D< T >::operator= | ( | const Point3D< T > & | p2 | ) | [inline] |
template<class T>
bool biu::Point3D< T >::operator== | ( | const Point3D< T > & | p2 | ) | const [inline] |
template<class T>
bool biu::Point3D< T >::operator> | ( | const Point3D< T > & | p2 | ) | const [inline] |
template<class T>
void biu::Point3D< T >::setX | ( | const T & | x_ | ) | [inline] |
template<class T>
void biu::Point3D< T >::setY | ( | const T & | y_ | ) | [inline] |
template<class T>
void biu::Point3D< T >::setZ | ( | const T & | z_ | ) | [inline] |
template<class T>
double biu::Point3D< T >::vectorLength | ( | ) | const [inline] |
Friends And Related Function Documentation
template<class T>
std::ostream& operator<< | ( | std::ostream & | out, | |
const Point3D< T > & | p | |||
) | [friend] |
Field Documentation
template<class T>
T biu::Point3D< T >::x [private] |
template<class T>
T biu::Point3D< T >::y [private] |
template<class T>
T biu::Point3D< T >::z [private] |
The documentation for this class was generated from the following file:
- src/biu/Point.hh