biu::NeighborVector Class Reference
#include <NeighborVector.hh>
Inheritance diagram for biu::NeighborVector:
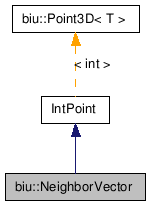
Detailed Description
A NeighborVector manages lattice specific neighborhood data. It handles the vector dependend move string and the related rotation matrix to generate relative move strings.
Definition at line 34 of file NeighborVector.hh.
Public Member Functions | |
double | distance (const Point3D &p2) const |
const Automorphism & | getAbs2RelRotation () const |
const Move & | getMove () const |
const Automorphism & | getRel2AbsRotation () const |
int | getX () const |
int | getY () const |
int | getZ () const |
bool | isEven () const |
NeighborVector (const IntPoint &point) | |
NeighborVector (const int x, const int y, const int z, const Move _move, const Automorphism &rel2absRot, const Automorphism &abs2relRot) | |
Point3D & | operator *= (const int &d) |
operator Point3D () const | |
operator std::vector () const | |
bool | operator!= (const Point3D &p2) const |
Point3D | operator+ (const Point3D &p2) const |
Point3D & | operator+= (const int &d) |
Point3D & | operator+= (const Point3D &p2) |
Point3D & | operator- () |
Point3D | operator- (const Point3D &p2) const |
Point3D & | operator-= (const int &d) |
Point3D & | operator-= (const Point3D &p2) |
Point3D & | operator/= (const int &d) |
bool | operator< (const Point3D &p2) const |
bool | operator== (const Point3D &p2) const |
bool | operator> (const Point3D &p2) const |
void | setX (const int &x_) |
void | setY (const int &y_) |
void | setZ (const int &z_) |
double | vectorLength () const |
virtual | ~NeighborVector () |
Private Attributes | |
Automorphism | abs2relRotation |
Move | move |
Automorphism | rel2absRotation |
Friends | |
std::ostream & | operator<< (std::ostream &out, const Point3D &p) |
Constructor & Destructor Documentation
biu::NeighborVector::NeighborVector | ( | const int | x, | |
const int | y, | |||
const int | z, | |||
const Move | _move, | |||
const Automorphism & | rel2absRot, | |||
const Automorphism & | abs2relRot | |||
) | [inline] |
Definition at line 46 of file NeighborVector.hh.
biu::NeighborVector::NeighborVector | ( | const IntPoint & | point | ) | [inline] |
Definition at line 54 of file NeighborVector.hh.
virtual biu::NeighborVector::~NeighborVector | ( | ) | [inline, virtual] |
Definition at line 57 of file NeighborVector.hh.
Member Function Documentation
double biu::Point3D< int >::distance | ( | const Point3D< int > & | p2 | ) | const [inline, inherited] |
const Automorphism& biu::NeighborVector::getAbs2RelRotation | ( | ) | const [inline] |
Definition at line 67 of file NeighborVector.hh.
const Move& biu::NeighborVector::getMove | ( | ) | const [inline] |
Definition at line 60 of file NeighborVector.hh.
const Automorphism& biu::NeighborVector::getRel2AbsRotation | ( | ) | const [inline] |
Definition at line 64 of file NeighborVector.hh.
int biu::Point3D< int >::getX | ( | ) | const [inline, inherited] |
int biu::Point3D< int >::getY | ( | ) | const [inline, inherited] |
int biu::Point3D< int >::getZ | ( | ) | const [inline, inherited] |
bool biu::Point3D< int >::isEven | ( | ) | const [inline, inherited] |
Point3D& biu::Point3D< int >::operator *= | ( | const int & | d | ) | [inline, inherited] |
biu::Point3D< int >::operator Point3D | ( | ) | const [inline, inherited] |
biu::Point3D< int >::operator std::vector | ( | ) | const [inline, inherited] |
bool biu::Point3D< int >::operator!= | ( | const Point3D< int > & | p2 | ) | const [inline, inherited] |
Point3D biu::Point3D< int >::operator+ | ( | const Point3D< int > & | p2 | ) | const [inline, inherited] |
Point3D& biu::Point3D< int >::operator+= | ( | const int & | d | ) | [inline, inherited] |
Point3D& biu::Point3D< int >::operator+= | ( | const Point3D< int > & | p2 | ) | [inline, inherited] |
Point3D& biu::Point3D< int >::operator- | ( | ) | [inline, inherited] |
Point3D biu::Point3D< int >::operator- | ( | const Point3D< int > & | p2 | ) | const [inline, inherited] |
Point3D& biu::Point3D< int >::operator-= | ( | const int & | d | ) | [inline, inherited] |
Point3D& biu::Point3D< int >::operator-= | ( | const Point3D< int > & | p2 | ) | [inline, inherited] |
Point3D& biu::Point3D< int >::operator/= | ( | const int & | d | ) | [inline, inherited] |
bool biu::Point3D< int >::operator< | ( | const Point3D< int > & | p2 | ) | const [inline, inherited] |
bool biu::Point3D< int >::operator== | ( | const Point3D< int > & | p2 | ) | const [inline, inherited] |
bool biu::Point3D< int >::operator> | ( | const Point3D< int > & | p2 | ) | const [inline, inherited] |
void biu::Point3D< int >::setX | ( | const int & | x_ | ) | [inline, inherited] |
void biu::Point3D< int >::setY | ( | const int & | y_ | ) | [inline, inherited] |
void biu::Point3D< int >::setZ | ( | const int & | z_ | ) | [inline, inherited] |
double biu::Point3D< int >::vectorLength | ( | ) | const [inline, inherited] |
Friends And Related Function Documentation
std::ostream& operator<< | ( | std::ostream & | out, | |
const Point3D< int > & | p | |||
) | [friend, inherited] |
Field Documentation
the rotation automorphism to convert absolute to relative moves
Definition at line 42 of file NeighborVector.hh.
Move biu::NeighborVector::move [private] |
the rotation automorphism to convert relative to absolute moves
Definition at line 39 of file NeighborVector.hh.
The documentation for this class was generated from the following file:
- src/biu/NeighborVector.hh