biu::LatticeFrame Class Reference
#include <LatticeFrame.hh>
Inheritance diagram for biu::LatticeFrame:
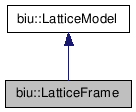
Detailed Description
A lattice frame is a subset of a lattice in which all points coordinates XYZ are in the range ZERO <= XYZ < FRAMESIZE.The lattice frame provides a one-to-one mapping of the managed points to an index and vice versa.
Definition at line 20 of file LatticeFrame.hh.
Public Types | |
typedef int | index_type |
Public Member Functions | |
virtual IPointVec | absMovesToPoints (const MoveSequence &absMoves) const |
MoveSequence | absMovesToRelMoves (const MoveSequence &absMoves) const |
virtual IntPoint | applyAbsMove (const IntPoint &actPoint, const Move &absMove) const |
virtual bool | areNeighbored (const IntPoint &first, const IntPoint &second) const |
virtual Move | getAbsMove (const IntPoint &lastPoint, const IntPoint &actPoint) const |
virtual IPointSet | getAllNeighPoints (const IntPoint ¢er) const |
IntPoint | getCenter () const |
LatticeDescriptor const *const | getDescriptor () const |
unsigned int | getFrameSize () const |
index_type | getIndex (const IntPoint &point) const |
std::vector< index_type > | getIndexedNeighborhood () const |
index_type | getMaxIndex () const |
const LatticeNeighborhood & | getNeighborhood () const |
IntPoint | getPoint (const index_type &index) const |
virtual std::string | getString (const MoveSequence &moveSeq) const |
MoveSequence | indicesToAbsMoves (const std::vector< index_type > indVec) const |
bool | isInFrame (const IntPoint &point) const |
LatticeFrame (const LatticeFrame &toCopy) | |
LatticeFrame (const LatticeDescriptor *const _latDescriptor, const unsigned int frameSize) | |
bool | operator!= (const LatticeModel &lm2) const |
bool | operator!= (const LatticeFrame &lf2) const |
bool | operator== (const LatticeModel &lm2) const |
bool | operator== (const LatticeFrame &lf2) const |
virtual MoveSequence | parseMoveString (const std::string &moveStr) const |
virtual MoveSequence | pointsToAbsMoves (const IPointVec &points) const |
virtual MoveSequence | pointsToRelMoves (const IPointVec &points) const |
MoveSequence | relMovesToAbsMoves (const MoveSequence &relMoves) const |
virtual IPointVec & | relMovesToPoints (const MoveSequence &relMoves, IPointVec &toFill) const |
virtual IPointVec | relMovesToPoints (const MoveSequence &relMoves) const |
void | setFrameSize (const unsigned int frameSize_) |
virtual | ~LatticeFrame () |
Protected Attributes | |
LatticeDescriptor const *const | latDescriptor |
const LatticeNeighborhood & | latNeighborhood |
Private Attributes | |
unsigned int | frameSize |
unsigned int | frameSizeP2 |
Member Typedef Documentation
typedef int biu::LatticeFrame::index_type |
Constructor & Destructor Documentation
biu::LatticeFrame::LatticeFrame | ( | const LatticeDescriptor *const | _latDescriptor, | |
const unsigned int | frameSize | |||
) |
construction
- Parameters:
-
_latDescriptor This lattice property handler has to be != NULL. frameSize the size of the lattice frame
Definition at line 8 of file LatticeFrame.cc.
biu::LatticeFrame::LatticeFrame | ( | const LatticeFrame & | toCopy | ) |
Definition at line 18 of file LatticeFrame.cc.
biu::LatticeFrame::~LatticeFrame | ( | ) | [virtual] |
Definition at line 25 of file LatticeFrame.cc.
Member Function Documentation
IPointVec biu::LatticeModel::absMovesToPoints | ( | const MoveSequence & | absMoves | ) | const [virtual, inherited] |
MoveSequence biu::LatticeModel::absMovesToRelMoves | ( | const MoveSequence & | absMoves | ) | const [inherited] |
Convert a sequence of absolute moves to a sequence of relative moves
Definition at line 54 of file LatticeModel.cc.
virtual bool biu::LatticeModel::areNeighbored | ( | const IntPoint & | first, | |
const IntPoint & | second | |||
) | const [virtual, inherited] |
Return whether or not two points in the lattice are neighbored, based on the current lattice descriptor.
- Parameters:
-
first the first point second the second point
- Returns:
- true if (second-first) is element of the neighborhood, false otherwise
virtual Move biu::LatticeModel::getAbsMove | ( | const IntPoint & | lastPoint, | |
const IntPoint & | actPoint | |||
) | const [virtual, inherited] |
Converts two points into an absolute move.
They have to be neighbored in the lattice.
IPointSet biu::LatticeModel::getAllNeighPoints | ( | const IntPoint & | center | ) | const [virtual, inherited] |
Calculates all neighbored points to the given center based on the current lattice descriptor.
Definition at line 171 of file LatticeModel.cc.
IntPoint biu::LatticeFrame::getCenter | ( | ) | const [inline] |
LatticeDescriptor const* const biu::LatticeModel::getDescriptor | ( | ) | const [inline, inherited] |
Returns a constant reference to the current lattice descriptor.
Definition at line 40 of file LatticeModel.hh.
unsigned int biu::LatticeFrame::getFrameSize | ( | ) | const [inline] |
LatticeFrame::index_type biu::LatticeFrame::getIndex | ( | const IntPoint & | point | ) | const |
Converts a point to its index. The Point has to be inside the frame borders (isInFrame() == true).
Definition at line 45 of file LatticeFrame.cc.
std::vector< LatticeFrame::index_type > biu::LatticeFrame::getIndexedNeighborhood | ( | ) | const |
Returns the indices of the neighbor vectors, this lattice frame is based on.
Definition at line 71 of file LatticeFrame.cc.
index_type biu::LatticeFrame::getMaxIndex | ( | ) | const [inline] |
Returns the highest index value available in this frame.
Definition at line 55 of file LatticeFrame.hh.
const LatticeNeighborhood& biu::LatticeModel::getNeighborhood | ( | ) | const [inline, inherited] |
IntPoint biu::LatticeFrame::getPoint | ( | const index_type & | index | ) | const |
Converts an index to its point coordinates. Assure that the index was created with the same frame size. Otherwise the convertion will lead to a differnt point.
Definition at line 50 of file LatticeFrame.cc.
virtual std::string biu::LatticeModel::getString | ( | const MoveSequence & | moveSeq | ) | const [virtual, inherited] |
Returns the string representation of the move sequence.
MoveSequence biu::LatticeFrame::indicesToAbsMoves | ( | const std::vector< index_type > | indVec | ) | const |
Converts a sequence of indices to the corresponding absolute move sequence.
Definition at line 80 of file LatticeFrame.cc.
bool biu::LatticeFrame::isInFrame | ( | const IntPoint & | point | ) | const |
bool biu::LatticeModel::operator!= | ( | const LatticeModel & | lm2 | ) | const [inherited] |
bool biu::LatticeFrame::operator!= | ( | const LatticeFrame & | lf2 | ) | const |
Definition at line 66 of file LatticeFrame.cc.
bool biu::LatticeModel::operator== | ( | const LatticeModel & | lm2 | ) | const [inherited] |
bool biu::LatticeFrame::operator== | ( | const LatticeFrame & | lf2 | ) | const |
Definition at line 58 of file LatticeFrame.cc.
virtual MoveSequence biu::LatticeModel::parseMoveString | ( | const std::string & | moveStr | ) | const [virtual, inherited] |
Converts an absolute/relative move string representation into an absolute/relative move sequence.
MoveSequence biu::LatticeModel::pointsToAbsMoves | ( | const IPointVec & | points | ) | const [virtual, inherited] |
Converts a vector of points to an absolute move string.
Consecutive positions have to be neighbored in the lattice!
Definition at line 158 of file LatticeModel.cc.
virtual MoveSequence biu::LatticeModel::pointsToRelMoves | ( | const IPointVec & | points | ) | const [virtual, inherited] |
Converts a vector of points to a relative move string.
Consecutive positions have to be neighbored in the lattice!
MoveSequence biu::LatticeModel::relMovesToAbsMoves | ( | const MoveSequence & | relMoves | ) | const [inherited] |
Convert a sequence of relative moves to a sequence of absolute moves
Definition at line 32 of file LatticeModel.cc.
IPointVec & biu::LatticeModel::relMovesToPoints | ( | const MoveSequence & | relMoves, | |
IPointVec & | toFill | |||
) | const [virtual, inherited] |
Converts a relative move string to coordinates written to the given vector toFill.
Definition at line 132 of file LatticeModel.cc.
IPointVec biu::LatticeModel::relMovesToPoints | ( | const MoveSequence & | relMoves | ) | const [virtual, inherited] |
void biu::LatticeFrame::setFrameSize | ( | const unsigned int | frameSize_ | ) |
Resizes the frame.
All indices, created with the old frame size will be incompatible with the new lattice frame!
Definition at line 30 of file LatticeFrame.cc.
Field Documentation
unsigned int biu::LatticeFrame::frameSize [private] |
unsigned int biu::LatticeFrame::frameSizeP2 [private] |
LatticeDescriptor const* const biu::LatticeModel::latDescriptor [protected, inherited] |
The lattice property handler (!= NULL).
Definition at line 25 of file LatticeModel.hh.
const LatticeNeighborhood& biu::LatticeModel::latNeighborhood [protected, inherited] |
The documentation for this class was generated from the following files:
- src/biu/LatticeFrame.hh
- src/biu/LatticeFrame.cc