cpsp::gecode::GC_ThreadingSpaceMoveDist Class Reference
#include <GC_ThreadingSpace.hh>
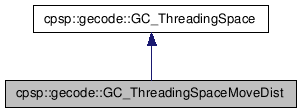
Detailed Description
CSP model that extends the CPSP approach so that resulting structures differ in a given number of movesDefinition at line 219 of file GC_ThreadingSpace.hh.
Public Types | |
typedef std::vector< unsigned int > | HullLevel |
enum | NeighPropLvl { CUSTOM_2, NO_PROP } |
typedef std::map< SeqFeatures, std::vector< unsigned int > > | SeqFeatureMap |
enum | SeqFeatures { P_SINGLET, H_SINGLET } |
typedef std::vector < Gecode::IntArgs > | SolutionVec |
Public Member Functions | |
virtual void | constrain (GC_ThreadingSpaceMoveDist *lastSolution) |
virtual SuperSpace * | copy (bool share) |
GC_ThreadingSpaceMoveDist (bool share, GC_ThreadingSpaceMoveDist &toCopy) | |
GC_ThreadingSpaceMoveDist (const std::string *sequence, const biu::LatticeFrame *latFrame, const biu::IndexVec *neighVecs, SeqFeatureMap *seqFeatureMap, const HullLevel *hullLvl, HCore *hCore, const GC_ThreadingSymmBreaker::GlobalShiftVec *shiftVec, int branchingType=BR_DFS|BR_SYM, SolutionVec *solMoves_=NULL, unsigned int maxEqual_=0) | |
virtual int | getIndex (const Gecode::VarBase *vb) const |
virtual int | getIndex (const Gecode::Int::IntView &v) const |
int | getRank (int index) const |
virtual biu::IndexVec | getSolution () const |
virtual void | handleSolution (GC_ThreadingSpace *solution) |
virtual void | handleSolution (GC_ThreadingSpaceMoveDist *solution) |
virtual void | print () const |
virtual | ~GC_ThreadingSpaceMoveDist () |
Data Fields | |
SolutionVec * | solMoves |
Protected Attributes | |
Gecode::IntVarArray | absMoves |
Gecode::IntVarArray | domains |
unsigned int | maxEqual |
std::vector< int > | rank |
Member Typedef Documentation
typedef std::vector<unsigned int> cpsp::gecode::GC_ThreadingSpace::HullLevel [inherited] |
Definition at line 75 of file GC_ThreadingSpace.hh.
typedef std::map< SeqFeatures, std::vector <unsigned int> > cpsp::gecode::GC_ThreadingSpace::SeqFeatureMap [inherited] |
Definition at line 73 of file GC_ThreadingSpace.hh.
typedef std::vector<Gecode::IntArgs> cpsp::gecode::GC_ThreadingSpaceMoveDist::SolutionVec |
Definition at line 222 of file GC_ThreadingSpace.hh.
Member Enumeration Documentation
enum cpsp::gecode::GC_ThreadingSpace::NeighPropLvl [inherited] |
neighborhood propagation level
- Enumerator:
-
CUSTOM_2 CUSTOM_2 : using an advanced binary propagator. NO_PROP NO_PROP : post no neighboring propagator.
Definition at line 78 of file GC_ThreadingSpace.hh.
enum cpsp::gecode::GC_ThreadingSpace::SeqFeatures [inherited] |
Constructor & Destructor Documentation
cpsp::gecode::GC_ThreadingSpaceMoveDist::GC_ThreadingSpaceMoveDist | ( | const std::string * | sequence, | |
const biu::LatticeFrame * | latFrame, | |||
const biu::IndexVec * | neighVecs, | |||
SeqFeatureMap * | seqFeatureMap, | |||
const HullLevel * | hullLvl, | |||
HCore * | hCore, | |||
const GC_ThreadingSymmBreaker::GlobalShiftVec * | shiftVec, | |||
int | branchingType = BR_DFS|BR_SYM , |
|||
SolutionVec * | solMoves_ = NULL , |
|||
unsigned int | maxEqual_ = 0 | |||
) | [inline] |
Definition at line 240 of file GC_ThreadingSpace.hh.
cpsp::gecode::GC_ThreadingSpaceMoveDist::GC_ThreadingSpaceMoveDist | ( | bool | share, | |
GC_ThreadingSpaceMoveDist & | toCopy | |||
) | [inline] |
Definition at line 279 of file GC_ThreadingSpace.hh.
virtual cpsp::gecode::GC_ThreadingSpaceMoveDist::~GC_ThreadingSpaceMoveDist | ( | ) | [inline, virtual] |
Definition at line 287 of file GC_ThreadingSpace.hh.
Member Function Documentation
virtual void cpsp::gecode::GC_ThreadingSpaceMoveDist::constrain | ( | GC_ThreadingSpaceMoveDist * | lastSolution | ) | [inline, virtual] |
adds additional constraints if BAB search is used to ensure a minimal position difference between the next and all other
Definition at line 295 of file GC_ThreadingSpace.hh.
virtual SuperSpace* cpsp::gecode::GC_ThreadingSpaceMoveDist::copy | ( | bool | share | ) | [inline, virtual] |
Reimplemented from cpsp::gecode::GC_ThreadingSpace.
Definition at line 290 of file GC_ThreadingSpace.hh.
virtual int cpsp::gecode::GC_ThreadingSpace::getIndex | ( | const Gecode::VarBase * | vb | ) | const [inline, virtual, inherited] |
Definition at line 129 of file GC_ThreadingSpace.hh.
virtual int cpsp::gecode::GC_ThreadingSpace::getIndex | ( | const Gecode::Int::IntView & | v | ) | const [inline, virtual, inherited] |
Definition at line 120 of file GC_ThreadingSpace.hh.
int cpsp::gecode::GC_ThreadingSpace::getRank | ( | int | index | ) | const [inherited] |
Definition at line 286 of file GC_ThreadingSpace.cc.
biu::IndexVec cpsp::gecode::GC_ThreadingSpace::getSolution | ( | ) | const [virtual, inherited] |
Returns a vector of indexed points thats a structure for the current CPSP.
Definition at line 271 of file GC_ThreadingSpace.cc.
virtual void cpsp::gecode::GC_ThreadingSpace::handleSolution | ( | GC_ThreadingSpace * | solution | ) | [inline, virtual, inherited] |
Definition at line 113 of file GC_ThreadingSpace.hh.
virtual void cpsp::gecode::GC_ThreadingSpaceMoveDist::handleSolution | ( | GC_ThreadingSpaceMoveDist * | solution | ) | [inline, virtual] |
handles the information of a solution to ensure the minimal distance of the next solution to all found
Definition at line 306 of file GC_ThreadingSpace.hh.
void cpsp::gecode::GC_ThreadingSpace::print | ( | void | ) | const [virtual, inherited] |
Definition at line 59 of file GC_ThreadingSpace.cc.
Field Documentation
Gecode::IntVarArray cpsp::gecode::GC_ThreadingSpaceMoveDist::absMoves [protected] |
Gecode::IntVarArray cpsp::gecode::GC_ThreadingSpace::domains [protected, inherited] |
unsigned int cpsp::gecode::GC_ThreadingSpaceMoveDist::maxEqual [protected] |
the maximal number of solution moves that may be equal to corresponding positions in already found solutions
Definition at line 230 of file GC_ThreadingSpace.hh.
std::vector<int> cpsp::gecode::GC_ThreadingSpace::rank [protected, inherited] |
Definition at line 89 of file GC_ThreadingSpace.hh.
The documentation for this class was generated from the following file:
- src/cpsp/gecode/GC_ThreadingSpace.hh