cpsp::gecode::GC_HPThreading Class Reference
#include <GC_HPThreading.hh>
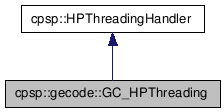
Detailed Description
This is a HPThreadingHandler implementation using the constraint propagation library Gecode(TM)Definition at line 44 of file GC_HPThreading.hh.
Public Types | |
enum | CORESELECTS { FIRST, ALL_BEST, ALL } |
enum | DISTANCE { DIST_POSITIONS, DIST_ABSMOVES, DIST_SHAPES, DIST_NONE } |
enum | RUNMODE { COUNT, ENUMERATE } |
Public Member Functions | |
unsigned int | countStructures (SuperSpace *space, unsigned int maxNum, bool useDDS=true, Gecode::Search::Statistics *statistic=NULL) |
virtual unsigned int | enumerateStructures (StringVec &structs) |
unsigned int | enumerateStructures (SuperSpace *space, unsigned int maxNum, Gecode::Search::Statistics *globalStat=NULL, std::ostream &out=std::cout) |
GC_HPThreading (const biu::LatticeDescriptor *latDescr_=NULL, HCoreDatabase *db=NULL, const std::string seq="") throw (cpsp::Exception) | |
virtual unsigned int | generateStructures () |
const unsigned int | getContactsInSeq (void) const |
HCoreDatabase * | getCoreDB (void) const |
const CORESELECTS | getCoreSelection (void) const |
const unsigned int | getCoreSize (void) const |
const unsigned int | getDistanceMin (void) const |
const DISTANCE | getDistanceMode (void) const |
const biu::LatticeDescriptor * | getLatticeDescriptor (void) const |
const unsigned int | getMaxStructures (void) const |
const bool | getNoOutput (void) const |
const bool | getNoRecomputation (void) const |
const bool | getNormalizeStructures (void) const |
const RUNMODE | getRunMode (void) const |
const std::string & | getSequence (void) |
const bool | getStatOutput (void) const |
const bool | getSymmetryBreaking (void) const |
const bool | getUsingDDS (void) const |
const bool | getVerboseOutput (void) const |
void | setCoreDB (HCoreDatabase *db) |
void | setCoreSelection (const CORESELECTS val) |
void | setDistanceMin (const unsigned int val) |
void | setDistanceMode (const DISTANCE val) |
void | setLatticeDescriptor (const biu::LatticeDescriptor *val) |
void | setMaxStructures (const unsigned int val) |
void | setNoOutput (const bool val) |
void | setNoRecomputation (const bool val) |
void | setNormalizeStructures (const bool val) |
void | setRunMode (const RUNMODE val) |
void | setSequence (const std::string &seq) throw (cpsp::Exception) |
void | setStatOutput (const bool val) |
void | setSymmetryBreaking (const bool val) |
void | setUsingDDS (const bool val) |
void | setVerboseOutput (const bool val) |
virtual | ~GC_HPThreading () |
Protected Member Functions | |
template<class Int, class SPACE> | |
Int | countBAB (SPACE *space, unsigned int c_d, unsigned int a_d, Gecode::Search::Statistics *stat, Gecode::Search::Stop *st, Int maxNum) |
template<class ENGINE, class SPACE> | |
unsigned int | enumerateStructures (SPACE *space, unsigned int maxNum, Gecode::Search::Statistics *stat, std::ostream &out) |
Protected Attributes | |
unsigned int | contactsInSeq |
HCoreDatabase * | coreDB |
CORESELECTS | coreSelection |
unsigned int | coreSize |
unsigned int | distanceMin |
DISTANCE | distanceMode |
GC_ThreadingSpace::HullLevel | hullLvl |
const biu::LatticeDescriptor * | latDescr |
biu::LatticeFrame * | latFrame |
unsigned int | maxPCoreDistance |
unsigned int | maxStructures |
unsigned int | minEvenOddHs |
bool | noOutput |
bool | noRecomputation |
bool | normalizeStructures |
RUNMODE | runMode |
GC_ThreadingSpace::SeqFeatureMap | seqFeatureMap |
std::string | sequence |
bool | statisticsOut |
bool | useDDS |
bool | useSymmetryBreaking |
bool | verboseOutput |
Member Enumeration Documentation
Constructor & Destructor Documentation
cpsp::gecode::GC_HPThreading::GC_HPThreading | ( | const biu::LatticeDescriptor * | latDescr_ = NULL , |
|
HCoreDatabase * | db = NULL , |
|||
const std::string | seq = "" | |||
) | throw (cpsp::Exception) |
cpsp::gecode::GC_HPThreading::~GC_HPThreading | ( | ) | [virtual] |
Definition at line 66 of file GC_HPThreading.cc.
Member Function Documentation
Int cpsp::gecode::GC_HPThreading::countBAB | ( | SPACE * | space, | |
unsigned int | c_d, | |||
unsigned int | a_d, | |||
Gecode::Search::Statistics * | stat, | |||
Gecode::Search::Stop * | st, | |||
Int | maxNum | |||
) | [inline, protected] |
Definition at line 317 of file GC_HPThreading.hh.
unsigned int cpsp::gecode::GC_HPThreading::countStructures | ( | SuperSpace * | space, | |
unsigned int | maxNum, | |||
bool | useDDS = true , |
|||
Gecode::Search::Statistics * | statistic = NULL | |||
) |
counts maxNum structures of space and returns their number
Definition at line 220 of file GC_HPThreading.cc.
unsigned int cpsp::gecode::GC_HPThreading::enumerateStructures | ( | StringVec & | structs | ) | [virtual] |
enumerates structures and writes their string representation into structs
Implements cpsp::HPThreadingHandler.
Definition at line 541 of file GC_HPThreading.cc.
unsigned int cpsp::gecode::GC_HPThreading::enumerateStructures | ( | SuperSpace * | space, | |
unsigned int | maxNum, | |||
Gecode::Search::Statistics * | globalStat = NULL , |
|||
std::ostream & | out = std::cout | |||
) |
enumerates maxNum structures of space and prints them to std::cout
enumerates structures and returns their number
Definition at line 174 of file GC_HPThreading.cc.
unsigned int cpsp::gecode::GC_HPThreading::enumerateStructures | ( | SPACE * | space, | |
unsigned int | maxNum, | |||
Gecode::Search::Statistics * | stat, | |||
std::ostream & | out | |||
) | [inline, protected] |
Definition at line 270 of file GC_HPThreading.hh.
unsigned int cpsp::gecode::GC_HPThreading::generateStructures | ( | ) | [virtual] |
starts structure generation with the internal parameters
- Returns:
- the number of generated structures
Implements cpsp::HPThreadingHandler.
Definition at line 308 of file GC_HPThreading.cc.
const unsigned int cpsp::gecode::GC_HPThreading::getContactsInSeq | ( | void | ) | const [inline] |
HCoreDatabase* cpsp::gecode::GC_HPThreading::getCoreDB | ( | void | ) | const [inline] |
const CORESELECTS cpsp::gecode::GC_HPThreading::getCoreSelection | ( | void | ) | const [inline] |
const unsigned int cpsp::gecode::GC_HPThreading::getCoreSize | ( | void | ) | const [inline] |
const unsigned int cpsp::gecode::GC_HPThreading::getDistanceMin | ( | void | ) | const [inline] |
const DISTANCE cpsp::gecode::GC_HPThreading::getDistanceMode | ( | void | ) | const [inline] |
const biu::LatticeDescriptor* cpsp::gecode::GC_HPThreading::getLatticeDescriptor | ( | void | ) | const [inline] |
const unsigned int cpsp::gecode::GC_HPThreading::getMaxStructures | ( | void | ) | const [inline] |
const bool cpsp::gecode::GC_HPThreading::getNoOutput | ( | void | ) | const [inline] |
const bool cpsp::gecode::GC_HPThreading::getNoRecomputation | ( | void | ) | const [inline] |
const bool cpsp::gecode::GC_HPThreading::getNormalizeStructures | ( | void | ) | const [inline] |
const RUNMODE cpsp::gecode::GC_HPThreading::getRunMode | ( | void | ) | const [inline] |
const std::string& cpsp::gecode::GC_HPThreading::getSequence | ( | void | ) | [inline] |
const bool cpsp::gecode::GC_HPThreading::getStatOutput | ( | void | ) | const [inline] |
const bool cpsp::gecode::GC_HPThreading::getSymmetryBreaking | ( | void | ) | const [inline] |
const bool cpsp::gecode::GC_HPThreading::getUsingDDS | ( | void | ) | const [inline] |
const bool cpsp::gecode::GC_HPThreading::getVerboseOutput | ( | void | ) | const [inline] |
void cpsp::gecode::GC_HPThreading::setCoreDB | ( | HCoreDatabase * | db | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setCoreSelection | ( | const CORESELECTS | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setDistanceMin | ( | const unsigned int | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setDistanceMode | ( | const DISTANCE | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setLatticeDescriptor | ( | const biu::LatticeDescriptor * | val | ) |
void cpsp::gecode::GC_HPThreading::setMaxStructures | ( | const unsigned int | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setNoOutput | ( | const bool | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setNoRecomputation | ( | const bool | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setNormalizeStructures | ( | const bool | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setRunMode | ( | const RUNMODE | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setSequence | ( | const std::string & | seq | ) | throw (cpsp::Exception) |
void cpsp::gecode::GC_HPThreading::setStatOutput | ( | const bool | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setSymmetryBreaking | ( | const bool | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setUsingDDS | ( | const bool | val | ) | [inline] |
void cpsp::gecode::GC_HPThreading::setVerboseOutput | ( | const bool | val | ) | [inline] |
Field Documentation
unsigned int cpsp::gecode::GC_HPThreading::contactsInSeq [protected] |
HCoreDatabase* cpsp::gecode::GC_HPThreading::coreDB [protected] |
unsigned int cpsp::gecode::GC_HPThreading::coreSize [protected] |
unsigned int cpsp::gecode::GC_HPThreading::distanceMin [protected] |
DISTANCE cpsp::gecode::GC_HPThreading::distanceMode [protected] |
const biu::LatticeDescriptor* cpsp::gecode::GC_HPThreading::latDescr [protected] |
the lattice descriptor of the lattice to do the threading in
Definition at line 63 of file GC_HPThreading.hh.
biu::LatticeFrame* cpsp::gecode::GC_HPThreading::latFrame [protected] |
unsigned int cpsp::gecode::GC_HPThreading::maxPCoreDistance [protected] |
unsigned int cpsp::gecode::GC_HPThreading::maxStructures [protected] |
unsigned int cpsp::gecode::GC_HPThreading::minEvenOddHs [protected] |
minimal even/odd distribution of core positions in the cubic# lattice
Definition at line 76 of file GC_HPThreading.hh.
bool cpsp::gecode::GC_HPThreading::noOutput [protected] |
whether or not generateStructures() does any output or not
Definition at line 94 of file GC_HPThreading.hh.
bool cpsp::gecode::GC_HPThreading::noRecomputation [protected] |
wether or not to run the search engine with or without batch recomputation during searhc (with == less propagations and more space consumption, see Gecode help)
Definition at line 100 of file GC_HPThreading.hh.
bool cpsp::gecode::GC_HPThreading::normalizeStructures [protected] |
RUNMODE cpsp::gecode::GC_HPThreading::runMode [protected] |
std::string cpsp::gecode::GC_HPThreading::sequence [protected] |
bool cpsp::gecode::GC_HPThreading::statisticsOut [protected] |
bool cpsp::gecode::GC_HPThreading::useDDS [protected] |
bool cpsp::gecode::GC_HPThreading::useSymmetryBreaking [protected] |
whether or not to take symmetric structures into account
Definition at line 84 of file GC_HPThreading.hh.
bool cpsp::gecode::GC_HPThreading::verboseOutput [protected] |
The documentation for this class was generated from the following files:
- src/cpsp/gecode/GC_HPThreading.hh
- src/cpsp/gecode/GC_HPThreading.cc